Your license is included in the applications you produce. This poses a potential security risk that someone could find the license and copy or leak it to others. Embedding the license as an internal resource protects it to a degree, but the original file can still be retrieved from the deployment package. This page includes code to help you protect your license file more securely by using encryption. Please choose one of the examples below:
C#
public void EncryptDecryptLicense()
{
string encryptedFilePath = MyDir + "EncryptedLicense.txt";
// Load the contents of the license into a byte array.
byte[] licBytes = File.ReadAllBytes("YourLicense.lic");
// Use this key only once for this license file.
// To protect another file first generate a new key.
byte[] key = GenerateKey(licBytes.Length);
// Write the encrypted license to disk.
File.WriteAllBytes(encryptedFilePath, EncryptDecryptLicense(licBytes, key));
// Load the encrypted license and decrypt it using the key.
byte[] decryptedLicense;
decryptedLicense = EncryptDecryptLicense(File.ReadAllBytes(encryptedFilePath), key);
// Load the decrypted license into a stream and set the license.
MemoryStream licenseStream = new MemoryStream(decryptedLicense);
License license = new License();
license.SetLicense(licenseStream);
}
/// <summary>
/// A method used for encrypting and decrypting data using XOR.
/// </summary>
public byte[] EncryptDecryptLicense(byte[] licBytes, byte[] key)
{
byte[] output = new byte[licBytes.Length];
for (int i = 0; i < licBytes.Length; i++)
output[i] = Convert.ToByte(licBytes[i] ^ key[i]);
return output;
}
/// <summary>
/// Generates a random key the same length as the license (a one time pad).
/// </summary>
public byte[] GenerateKey(long size)
{
RNGCryptoServiceProvider rng = new RNGCryptoServiceProvider();
byte[] strongBytes = new Byte[size];
rng.GetBytes(strongBytes);
return strongBytes;
}
Java
public void encryptDecryptLicense() throws Exception
{
String encryptedFilePath = getMyDir + "EncryptedLicense.txt";
// Load the contents of the license into a byte array.
byte[] licBytes = readAllBytesFromFile("YourLicense.lic");
// Use this key only once for this license file.
//To protect another file first generate a new key.
byte[] key = generateKey(licBytes.Length);
// Write the encrypted license to disk.
BufferedOutputStream output = new BufferedOutputStream(new FileOutputStream(
encryptedFilePath));
output.write(encryptDecryptLicese(licBytes, key));
output.close();
// Load the encrypted license and decrypt it using the key.
byte[] decryptedLicense = encryptDecryptLicense(
readAllBytesFromFile(encryptedFilePath), key);
// Load the decrypted license into a stream and set the license.
ByteArrayInputStream licenseStream = new ByteArrayInputStream(decryptedLicense);
License license = new License();
license.SetLicense(licenseStream);
}
// A method used for encrypting and decrypting data using XOR.
public byte[] encryptDecryptLicense(byte[] licBytes, byte[] key)
{
byte[] output = new byte[licBytes.Length];
for (int i = 0; i < licBytes.Length; i++)
output[i] = (byte)(licBytes[i] ^ key[i]);
return output;
}
// Generates a random key the same length as the license (a one time pad).
public byte[] generateKey(int size)
{
SecureRandom rng = new SecureRandom();
byte[] strongBytes = new byte[size];
rng.nextBytes(strongBytes);
return strongBytes;
}
public byte[] readAllBytesFromFile(String filePath) Throws IOException
{
int pos;
InputStream inputStream = new FileInputStream(filePath);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
while ((pos = inputStream.Read() != -1)
bos.write(pos);
inputStream.close();
return bos.toByteArray();
}
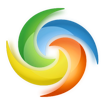
Questions?
If you have any questions or problems, please feel free to contact our sales support who will be glad to assist.